Qt Slot Call Order
I have a doubt about the call order of slots. If I connect a single signal to more than one object/slot, the calls to the slot functions will follow the same order as they were connected? For example: QObject::connect ( this, SIGNAL(valueChanged(int)), object1, SLOT(slot1(int)) ). In PyQt, connection between a signal and a slot can be achieved in different ways. Following are most commonly used techniques − QtCore.QObject.connect(widget, QtCore.SIGNAL(‘signalname’), slotfunction) A more convenient way to call a slotfunction, when a signal is emitted by a widget is as follows − widget.signal.connect(slotfunction). The following simple code snippet shows how to create and use QPushButton. It has been tested on Qt Symbian Simulator. An instance of QPushButton is created. Signal released is connected to slot handleButton which changes the text and the size of the button. To build and run the example: Create an empty folder. In Qt v4.5 and earlier: No, the order is undefined as can be seen in the documentation here: If several slots are connected to one signal, the slots will be executed one after the other, in an arbitrary order, when the signal is emitted. Edit: From version 4.6 onwards this is no longer true. Now the slots will run in the order they are connected.
As Qt WebKit is replaced by Qt WebEngine(you can refer to this post about porting issues), accessing html elements from C++ directly becomes impossible. Many works originally done by QWebKit classes are now transferred to javascript. Javascript is used to manipulate web content. And you need to call runJavaScript from C++ code to run javascript on the web page loaded by QWebEngineView.To get web elements, a communication mechanism is invented to bridge the C++ world and the javascript world. The bridging mechanism is more than obtaining the values of web page elements. It provides the ways in which C++ code can call javascript functions, and javascript can invoke C++ functions as well.The values of variables can also be passed from C++ to javascript, and vice versa. Let’s consider the following application scenarios:
javascript code calls C++ function
C++ code should provide a class which contains the to-be-called function(as a slot), then register an object of this class to a QWebChannel object, and set the web channel object to the web page object in the QWebEngineView
To invoke the C++ function jscallme, javascript should new an instance of QWebChannel object.
QWebChannel is defined in qwebchannel.js(you can find this file in the example folder of Qt installation directory) so the script should be loaded first. In the function passed as the second parameter of function QWebChannel, the exposed object from C++ world(webobj in C++) channel.objects.webobj is assigned to the javascript variable webobj, and then assigned to window.foo so you can use foo elsewhere to refer to the object. After the function is executed, javascript can call the C++ slot function jscallme as follows:
Pass data from javascript to C++
We’ve known how to call C++ function from javascript. You should be able to figure out a way to pass data from javascript to C++, i.e., as parameter(s) of function. We re-implement jscallme as follows:
, and invoking of the function from js would be:
Note that the “const” before the parameter can not be omitted, otherwise, you will get the following error:
Could not convert argument QJsonValue(string, “sd”) to target type .
Although data can be passed as parameters of function, it would be more convenient if we can pass data by setting an attribute of an object like:
We expect after the code is executed, “somedata” will be stored in a member variable of the exposed object (webobj) in C++. This is done by delaring a qt property in C++ class:
Now if you execute foo.someattribute=”somedata” in javascript, m_someattribute in C++ will be “somedata”.
Pass data from C++ to javascript
We can send data from C++ to javascript using signals. We emit a signal with the data to send as the parameter of the signal. Javascript must connect the signal to a function to receive the data.
The line “webobj.someattributeChanged.connect(updateattribute)” connects the C++ signal someattributeChanged to the javascript function updateattribute. Note that although updateattribute takes one parameter “text”, we did not provide the parameter value in connect. In fact, we do not know the parameter value passed to updateattribute until the signal is received. The signal is accompanied by a parameter “attr” which is passed as the “text” parameter of updateattribute. Now, if you call webobj->setsomeattribute(“hello”), you will see the value of the html element with id “#attrid” is set to “hello”. Note that although we connect the member m_someattribute to the qt property someattribute in the above example, it is not a required step. The signal mechanism alone can realize the delivery of data.
We can make things even simpler by adding the NOTIFY parameter when declaring the someattribute property.
Now, if you call webobj->setProperty(“someattribute”,”hello”) somewhere in C++, the signal “someattributeChanged” is automatically emitted and our web page gets updated.
C++ invokes javascript function
This is much straightforward compared with invoking C++ function from js. Just use runJavaScript passing the function as the parameter as follows:
It assumes the jsfun is already defined on your web page, otherwise, you have to define it in the string parameter. The return value is asynchronously passed to the lambda expression as the parameter v.
Now, back to the question raised at the beginning of the post: How to get the value of an html element in C++? It can be done as follows:
It uses jQuery functions so make sure jQuery lib is running on your web page.
EnArBgDeElEsFaFiFrHiHuItJaKnKoMsNlPlPtRuSqThTrUkZh
This page was used to describe the new signal and slot syntax during its development. The feature is now released with Qt 5.
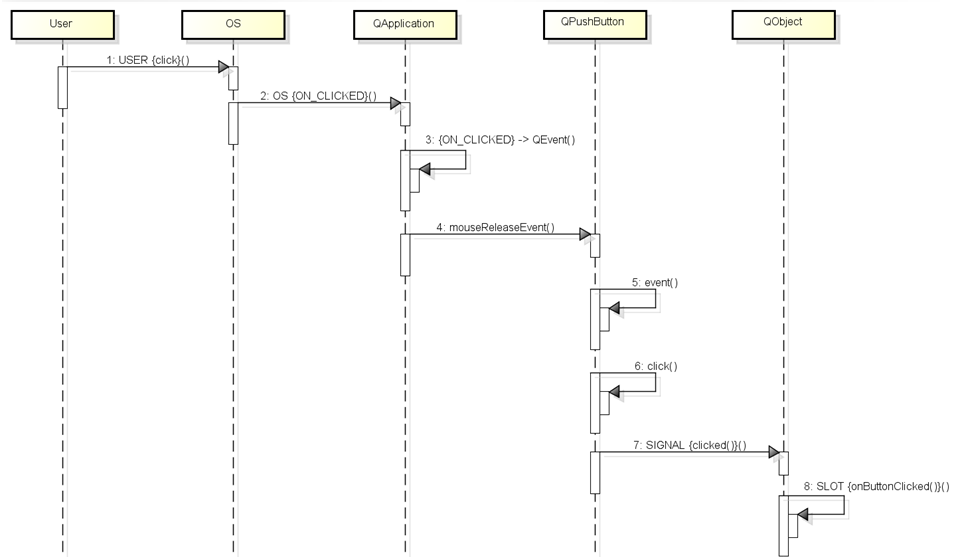
- Differences between String-Based and Functor-Based Connections (Official documentation)
- Introduction (Woboq blog)
- Implementation Details (Woboq blog)
Note: This is in addition to the old string-based syntax which remains valid.
- 1Connecting in Qt 5
- 2Disconnecting in Qt 5
- 4Error reporting
- 5Open questions
Connecting in Qt 5
There are several ways to connect a signal in Qt 5.
Old syntax
Qt 5 continues to support the old string-based syntax for connecting signals and slots defined in a QObject or any class that inherits from QObject (including QWidget)

New: connecting to QObject member
Here's Qt 5's new way to connect two QObjects and pass non-string objects:
Qt Signal Slot Call Order
Pros
- Compile time check of the existence of the signals and slot, of the types, or if the Q_OBJECT is missing.
- Argument can be by typedefs or with different namespace specifier, and it works.
- Possibility to automatically cast the types if there is implicit conversion (e.g. from QString to QVariant)
- It is possible to connect to any member function of QObject, not only slots.
Cons
- More complicated syntax? (you need to specify the type of your object)
- Very complicated syntax in cases of overloads? (see below)
- Default arguments in slot is not supported anymore.
New: connecting to simple function
The new syntax can even connect to functions, not just QObjects:
Pros
- Can be used with std::bind:
- Can be used with C++11 lambda expressions:
Cons
- There is no automatic disconnection when the 'receiver' is destroyed because it's a functor with no QObject. However, since 5.2 there is an overload which adds a 'context object'. When that object is destroyed, the connection is broken (the context is also used for the thread affinity: the lambda will be called in the thread of the event loop of the object used as context).
Disconnecting in Qt 5
As you might expect, there are some changes in how connections can be terminated in Qt 5, too.
Old way
You can disconnect in the old way (using SIGNAL, SLOT) but only if
- You connected using the old way, or
- If you want to disconnect all the slots from a given signal using wild card character
Symetric to the function pointer one
Only works if you connected with the symmetric call, with function pointers (Or you can also use 0 for wild card)In particular, does not work with static function, functors or lambda functions.
New way using QMetaObject::Connection
Works in all cases, including lambda functions or functors.
Asynchronous made easier
With C++11 it is possible to keep the code inline
Here's a QDialog without re-entering the eventloop, and keeping the code where it belongs:
Another example using QHttpServer : http://pastebin.com/pfbTMqUm
Error reporting
Tested with GCC.
Fortunately, IDEs like Qt Creator simplifies the function naming
Missing Q_OBJECT in class definition
Qt Slot Call Orders
Type mismatch
Open questions
Default arguments in slot
If you have code like this:
The old method allows you to connect that slot to a signal that does not have arguments.But I cannot know with template code if a function has default arguments or not.So this feature is disabled.
There was an implementation that falls back to the old method if there are more arguments in the slot than in the signal.This however is quite inconsistent, since the old method does not perform type-checking or type conversion. It was removed from the patch that has been merged.
Overload
As you might see in the example above, connecting to QAbstractSocket::error is not really beautiful since error has an overload, and taking the address of an overloaded function requires explicit casting, e.g. a connection that previously was made as follows:
connect(mySpinBox, SIGNAL(valueChanged(int)), mySlider, SLOT(setValue(int));
cannot be simply converted to:
...because QSpinBox has two signals named valueChanged() with different arguments. Instead, the new code needs to be:
Unfortunately, using an explicit cast here allows several types of errors to slip past the compiler. Adding a temporary variable assignment preserves these compile-time checks:
Some macro could help (with C++11 or typeof extensions). A template based solution was introduced in Qt 5.7: qOverload
The best thing is probably to recommend not to overload signals or slots …
… but we have been adding overloads in past minor releases of Qt because taking the address of a function was not a use case we support. But now this would be impossible without breaking the source compatibility.
Qt Slot Call Ordering
Disconnect
Should QMetaObject::Connection have a disconnect() function?
The other problem is that there is no automatic disconnection for some object in the closure if we use the syntax that takes a closure.One could add a list of objects in the disconnection, or a new function like QMetaObject::Connection::require
Callbacks
Function such as QHostInfo::lookupHost or QTimer::singleShot or QFileDialog::open take a QObject receiver and char* slot.This does not work for the new method.If one wants to do callback C++ way, one should use std::functionBut we cannot use STL types in our ABI, so a QFunction should be done to copy std::function.In any case, this is irrelevant for QObject connections.