Poker Program Java
- The Poker hand is passed in explicitly using the parameter variable Card h Rationale for making sine, cosine, etc as class methods You can now understand why Java define the methods sin, cos, etc. As class methods Input (s) of the sin, cos, etc.
- This is the #1 poker tracking program and HUD available today in my opinion. By the way, if you don't know what a poker HUD is, read this article of mine first where I explain it all. A good poker tracking program and HUD is absolutely essential poker software for serious poker players these days.
Write methods that test for the other poker hands. Some are easier than others. You might find it useful to write some general-purpose helper methods that can be used for more than one test. In some poker games, players get seven cards each, and they form a hand with the best five of the seven. Modify your program to generate seven-card hands. Need help with Java poker dice project. Must use the methods provided. Can't get it to run, not sure what to fix. For this lab you will write a Java program that plays the game Poker Dice. In this game, five dice are rolled and scored as if they were a hand of playing cards. The game goes through two separate phases.
There are different ways of doing the same thing, especially when you are programming. In Java, there are several ways to do the same thing, and in this tutorial, various techniques have been demonstrated to calculate the power of a number.
Required Knowledge

To understand this lesson, the level of difficulty is low, but the basic understanding of Java arithmetic operators, data types, basic input/output and loop is necessary.
Used Techniques
In Java, three techniques are used primarily to find the power of any number. These are:
- Calculate the power of a number through while loop.
- Calculate the power of a number by through for loop.
- Calculate the power of a number by through pow() function.
To calculate the power of any number, the base number and an exponent are required.
For the numerical input value, you can use predefined standard values, or take input from the user through scanner class, or take it through command line arguments.
Calculating the Power of a Number Through the While Loop in Java
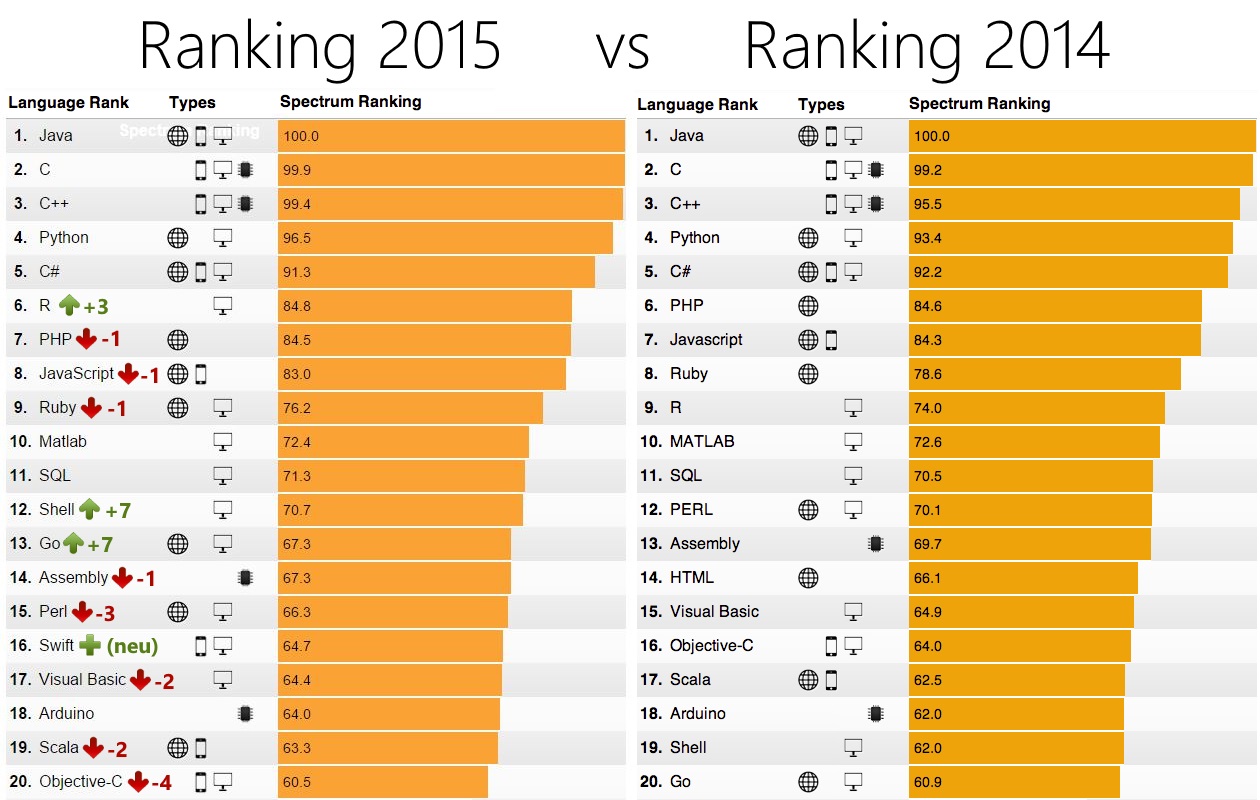

- In the above program, the base number and exponent values have been assigned 2 and 3, respectively.
- Using While Loop we keep multiplying temp by besanumber until the exponent becomes zero.
- We have multiplied temp by basenumber three times, hence the result would be = 1 * 2 * 2 * 2 = 8.
Calculating the Power of a Number Through the For Loop in Java
- In the above program, we used for loop instead of while loop, and the rest of the programmatic logic is the same.
Calculate the Power of a Number by Through pow() function
- Above program used Math.pow() function and it's also capable of working with a negative exponent.
Introduction: How to Make a Poker Game in Java
This instructable is for those that already some what know Java and want to create a game of poker within Java. First of all, you will need a computer with some sort of coding application or website that allows the use of Java. I recommend using DrJava or BlueJ. If you are not able to use an application like those two then I would recommend using the website repl.it. Once you have a Java application or website you are ready to start coding you poker program.
Step 1: Create a Deck of Cards
The first thing that you need to do to be able to create a game of poker in Java is to create a deck of cards. To do this create two public static methods, one that determines a random suit, and the other determining a random number from two to fourteen. In your main method create an array that will hold all fifty two cards. Use an array to place all fifty two cards in the array. Before putting the card in the array use a for loop to make sure that the card isn't already in the array of fifty two cards. If the card isn't in the array already then place it in the array. Once the array is filled with all fifty two cards creating a shuffled deck you can then move onto the next step.
Step 2: Give the Player Five Random Cards From the Deck
The next step is to give the player five random cards from your shuffled deck. To give the player five random cards you need to use a for loop and use a random number from zero to fifty one using Math.random. Use the for loop and the random number to choose a random card from your shuffled deck of cards. After choosing five random cards print them to allow the player to see what cards they have. You are now ready to move on to the next step.
Step 3: Create for Loops, If Statements, and While Loops to Determine What Combination the Player Has
You are now ready for the third step of the process to create a game of poker in Java. The third step is to use for loops, if statements, and while loops to tell the player what combination they have. You will want to start with the royal flush. Use a for loop and two if statements to determine if the player has a royal flush or not. Using the for loop you will determine if all the cards have the same suit, and then using the two if statements you will determine if the cards are a ten, Jack, Queen, King, and an ace. After the royal flush you will use two while loops and three if statements to determine if the player has a straight flush. Next will be four of a kind, and you will use a while loop and three if statements to determine if they have four of a kind. After four of a kind is full house. You will use a single if statement to determine a full house. After a full house is the flush where you will use a while loop and an if statement. After a flush you will use a while loop and two if statements for both straights and three of a kind. You will then use a while loop and an if statement for both two pairs and two of a kind. Finally you will only need one if statement to determine if the player has just a high card. Now it is time to move onto the last and final step.
Step 4: Tell the Player What Their Combination Is
The final step is to tell the player what their combination is. To tell them their combination you will use println and System.exit() within the loops and statements within the the combination's code. The println will tell the player what their combination is, and the System.exit() will end the program.
Be the First to Share
Recommendations
Poker Dice Java Program
Poker Game Java Program
Anything Goes Contest
Make it Real Student Design Challenge
Block Code Contest